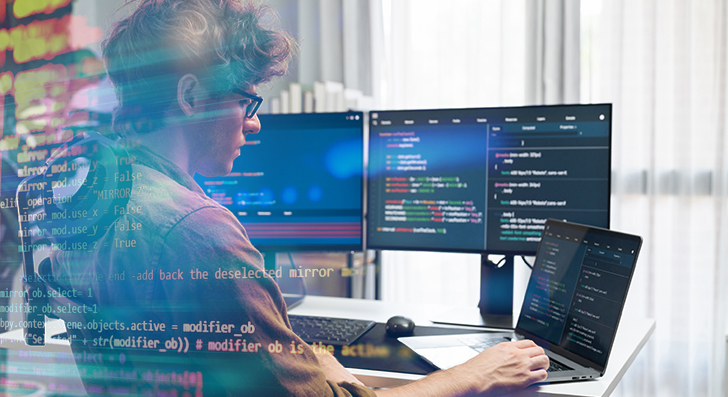
Scalability implies your software can take care of progress—much more users, extra knowledge, and a lot more site visitors—devoid of breaking. Being a developer, creating with scalability in your mind will save time and tension afterwards. Listed here’s a transparent and functional manual that can assist you begin by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a little something you bolt on later on—it ought to be portion of your plan from the start. Many apps are unsuccessful whenever they grow rapidly because the initial design and style can’t handle the extra load. For a developer, you have to Assume early about how your technique will behave stressed.
Begin by coming up with your architecture to get adaptable. Stay away from monolithic codebases wherever every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into scaled-down, unbiased components. Every single module or company can scale on its own devoid of influencing the whole method.
Also, think about your database from day one particular. Will it have to have to handle a million consumers or maybe 100? Pick the proper variety—relational or NoSQL—based upon how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them still.
A further important level is to stop hardcoding assumptions. Don’t produce code that only is effective less than current conditions. Consider what would occur Should your user foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that assist scaling, like information queues or event-driven systems. These enable your application cope with additional requests devoid of finding overloaded.
Any time you Make with scalability in mind, you're not just preparing for success—you are decreasing potential head aches. A nicely-planned system is easier to take care of, adapt, and improve. It’s greater to arrange early than to rebuild afterwards.
Use the best Database
Choosing the ideal databases is actually a important part of building scalable applications. Not all databases are built the same, and utilizing the Improper one can gradual you down or maybe lead to failures as your application grows.
Start off by comprehending your knowledge. Is it really structured, like rows in the table? If Certainly, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically robust with interactions, transactions, and regularity. Additionally they assistance scaling procedures like read through replicas, indexing, and partitioning to deal with extra website traffic and info.
If your knowledge is a lot more flexible—like person activity logs, products catalogs, or paperwork—think about a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with large volumes of unstructured or semi-structured knowledge and may scale horizontally much more quickly.
Also, contemplate your browse and produce designs. Are you executing lots of reads with less writes? Use caching and skim replicas. Will you be handling a significant write load? Explore databases which will take care of superior compose throughput, or simply event-primarily based info storage devices like Apache Kafka (for short term details streams).
It’s also wise to Assume in advance. You might not require Innovative scaling options now, but deciding on a database that supports them suggests you received’t need to switch later on.
Use indexing to hurry up queries. Stay clear of pointless joins. Normalize or denormalize your facts depending on your accessibility patterns. And usually watch databases general performance when you develop.
In brief, the correct database depends upon your app’s structure, speed needs, And the way you count on it to expand. Consider time to pick wisely—it’ll help you save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, each and every tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Make successful logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove anything avoidable. Don’t select the most complicated solution if a straightforward a single performs. Keep your capabilities quick, focused, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take also long to operate or employs an excessive amount of memory.
Upcoming, examine your databases queries. These usually gradual items down more than the code by itself. Make sure Every single question only asks for the info you actually will need. Steer clear of Pick out *, which fetches every thing, and as a substitute select distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, Specially across massive tables.
If you recognize a similar information currently being asked for again and again, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t should repeat expensive operations.
Also, batch your database operations after you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with large datasets. Code and queries that function wonderful with one hundred data could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These steps assist your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and much more site visitors. If every little thing goes by a person server, it will eventually immediately turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across a number of servers. As an alternative to a single server carrying out each of the perform, the load balancer routes customers to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to setup.
Caching is about storing data quickly so it may be reused quickly. When people request the same facts once again—like an item site or even a profile—you don’t must fetch it from the databases each time. You could serve it within the cache.
There are 2 common sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quick obtain.
2. Client-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down database load, enhances speed, and can make your application a lot more economical.
Use caching for things that don’t improve frequently. And generally be sure your cache is updated when info does improve.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application tackle much more people, continue to be quick, and Get well from complications. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To build scalable applications, you may need instruments that permit your application develop very easily. That’s wherever cloud platforms and containers are available. They give you flexibility, decrease setup time, and make scaling A great deal smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you'll need them. You don’t must acquire hardware or guess foreseeable future ability. When website traffic boosts, you could increase extra sources with only a few clicks or instantly employing automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your app in lieu of running infrastructure.
Containers are A different critical Software. A container offers your app and every thing it has to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
When your app uses various containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If 1 part of your respective app crashes, it restarts it quickly.
Containers also ensure it is easy to different elements of your application into companies. You are able to update or scale sections independently, which can be perfect for general performance and dependability.
To put it briefly, employing cloud and container tools signifies you are able to scale rapid, deploy effortlessly, and Get well rapidly when challenges occur. In order for you your app to increase without the need of limitations, get started utilizing these instruments early. They save time, cut down danger, and make it easier to stay focused on constructing, not correcting.
Keep track of Anything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is executing, location challenges early, and make much better choices as your application grows. It’s a key Portion of constructing scalable systems.
Begin by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just keep track of your servers—keep track here of your app also. Keep watch over just how long it requires for buyers to load internet pages, how frequently faults materialize, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening inside your code.
Set up alerts for important problems. For example, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair problems quickly, frequently prior to users even see.
Checking is additionally helpful when you make variations. When you deploy a whole new characteristic and see a spike in faults or slowdowns, it is possible to roll it back right before it will cause actual harm.
As your application grows, targeted traffic and information maximize. Devoid of monitoring, you’ll pass up signs of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about comprehending your procedure and ensuring it really works effectively, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid Basis. By designing meticulously, optimizing wisely, and using the ideal equipment, you could Construct applications that develop efficiently without breaking under pressure. Start out small, Consider significant, and Develop sensible.